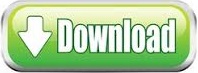
Np.mgrid and dense/fleshed out grids > yy, xx = np.mgridĪrray(, Plt.scatter(np.zeros_like(yy), yy, color="red", marker="x") Plt.scatter(xx, np.zeros_like(xx), color="blue", marker="*") This already looks like coordinates, specifically the x and y lines for 2D plots. np.ogrid and sparse grids > import numpy as npĪrray(])Īs already said the output is reversed when compared to np.meshgrid, that's why I unpacked it as yy, xx instead of xx, yy: > xx, yy = np.meshgrid(np.arange(-5, 6), np.arange(-5, 6), sparse=True) The other way around for np.mgrid and np.ogrid. Often this doesn't matter but you should give meaningful variable names depending on the context.įor example, in case of a 2D grid and it makes sense to name the first returned item of np.meshgrid x and the second one y while it's Np.meshgrid and np.ogrid and np.mgrid: The first two returned values (if there are two or more) are reversed. Note that there is a significant difference between Here np.mgrid represents the sparse=False and np.ogrid the sparse=True case (I refer to the sparse argument of np.meshgrid). However, np.meshgrid itself isn't often used directly, mostly one just uses one of similar objects np.mgrid or np.ogrid. That makes it much easier to keep track of coordinates and (even more importantly) you can pass them to functions that need to know the coordinates. So if you have two indices, let's say x and y (that's why the return value of meshgrid is usually xx or xs instead of x in this case I chose h for horizontally!) then you can get the x coordinate of the point, the y coordinate of the point and the value at that point by using: h # horizontal coordinate Suppose your origin is the upper left corner and you want arrays that represent the distance you could use: import numpy as np However, each value represents a 3 x 2 kilometer area (horizontal x vertical). However when that's not the case you somehow need to store coordinates alongside your data. Then you can just use the indices of an array as the index. The reason you need coordinate matrices with Python/NumPy is that there is no direct relation from coordinates to values, except when your coordinates start with zero and are purely positive integers. You probably just asked yourself: Why do we need to create coordinate matrices? So it's primary purpose is to create a coordinates matrices. Make N-D coordinate arrays for vectorized evaluations of N-D scalar/vector fields over N-D grids, given one-dimensional coordinate arrays x1, x2., xn. Return coordinate matrices from coordinate vectors. contourf)Īctually the purpose of np.meshgrid is already mentioned in the documentation: In the example that you have provided in your post, it is simply a way to sample a function ( sin(x**2 + y**2) / (x**2 + y**2)) over a range of values for x and y.īecause this function has been sampled on a rectangular grid, the function can now be visualized as an "image".Īdditionally, the result can now be passed to functions which expect data on rectangular grid (i.e. Plt.plot(xx, yy, marker='.', color='k', linestyle='none')Ĭreation of these rectangular grids is useful for a number of tasks.
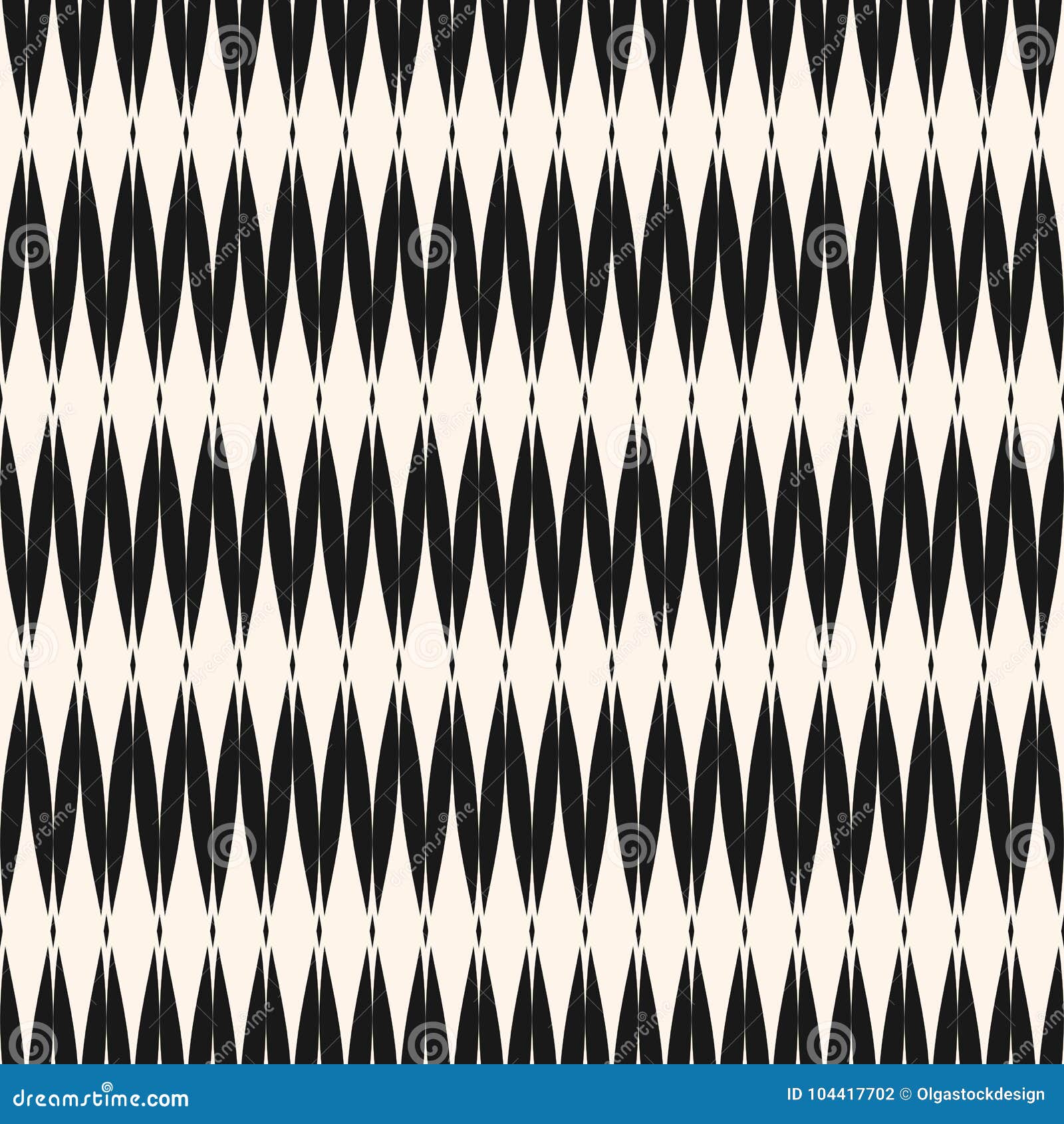
Now, when we call meshgrid, we get the previous output automatically. Instead, meshgrid can actually generate this for us: all we have to specify are the unique x and y values. Obviously, this gets very tedious especially for large ranges of x and y. We can then plot these to verify that they are a grid: plt.plot(x,y, marker='.', color='k', linestyle='none') This would result in the following x and y matrices, such that the pairing of the corresponding element in each matrix gives the x and y coordinates of a point in the grid.
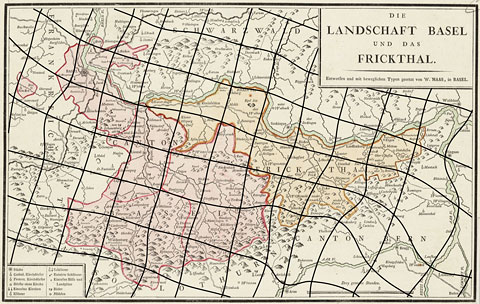
This is going to be 25 points, right? So if we wanted to create an x and y array for all of these points, we could do the following. To create a rectangular grid, we need every combination of the x and y points. So, for example, if we want to create a grid where we have a point at each integer value between 0 and 4 in both the x and y directions. The purpose of meshgrid is to create a rectangular grid out of an array of x values and an array of y values.
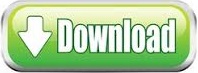